[et_pb_section][et_pb_row][et_pb_column type=”2_3″][et_pb_text admin_label=”Text”]
[box type=”info”] Before you do this tutorial you should go through Parts 1-3. Be super careful to read the instructions here before you change code. Where the code goes is just as important as the code itself. [/box]
There are three pieces of code we’re going to add to our project in order to get the bricks to appear and then disappear when we hit them with the ball.
- First, we’ll create the bricks in the setup part of our code. As we add them, we’ll put them in a list.
2. Then, we’ll draw all the bricks. Since they are in a list, we can use a for … in loop to draw them all.
3. Finally, we’ll use another for … in loop to check for collisions between the ball and the bricks. When the ball hits a brick, we’ll remove it from the list.
Creating the Bricks
Figuring out where to put our bricks is the hard part. We have a window that is 800 pixels wide. We’ll put ten bricks across, with each brick 60 pixels wide and 20 pixels tall. We’ll leave 20 pixels of space between the bricks, and 10 pixels at each end.
Vertically, we’ll make three rows of bricks, with 60 pixels empty at the top and 20 pixels between the rows.
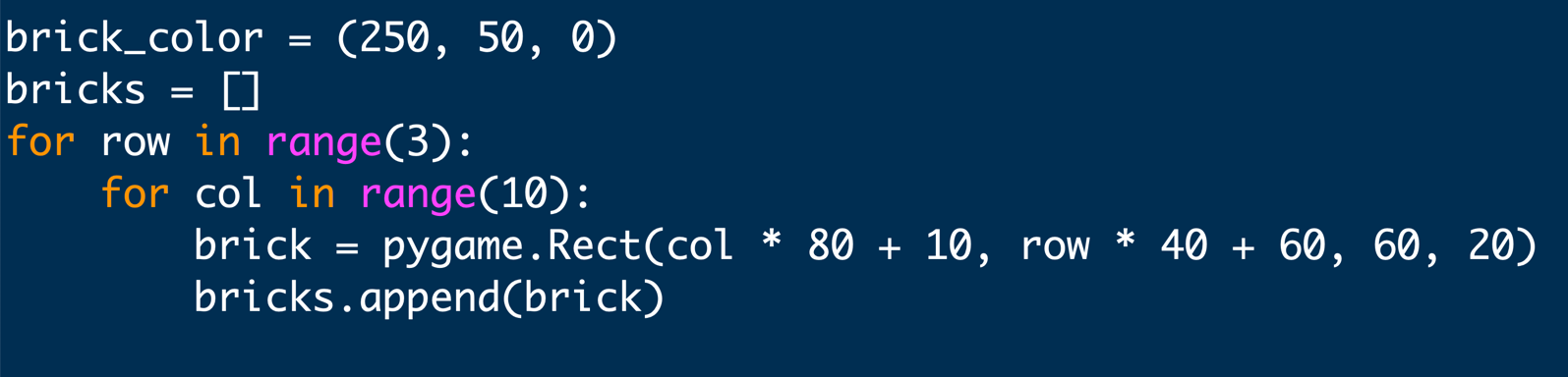
The brick variable is created and used 30 times, but be careful – our list is called bricks, and that is what we have to use in the other parts of the program.
You can (and should) run your program now to make sure it doesn’t crash. But you won’t see any bricks.
Drawing the Bricks
Down in the drawing part of the program, we draw all the bricks. Again we’ll use the brick variable, but this one doesn’t have anything to do with the one in setup. Its just a temporary name for each brick while we draw it.

Now when you run it, you should see all the bricks.
Brick Collisions
Next, we’ll delete bricks when the ball hits them, and reverse the direction of the ball.
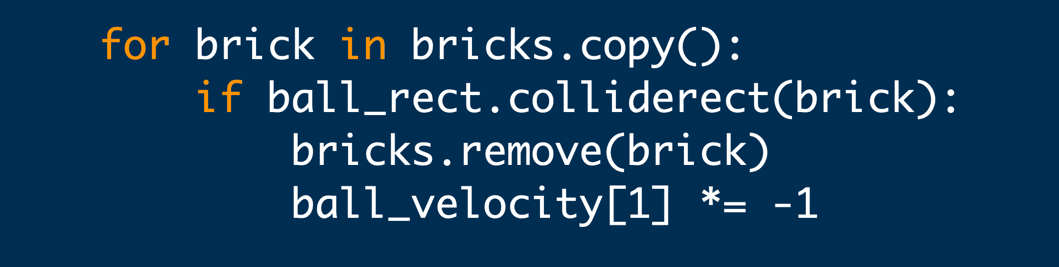
Why did we write bricks.copy()? In the block of code in the for .. in loop, we sometimes change bricks by removing an element. Because of this we need to make a copy of bricks and iterate over that. If you don’t you will see some strange behavior once in a while, where the brick that gets deleted is different from the one hit. The basic rule is don’t change the list you’re iterating over. And so we make a copy and iterate over that.
The game should basically work. In the next part of the tutorial, we’ll add some text to the screen that tells the player how many bricks are left.
Next: Pygame Tutorial Part 5: Score
[/et_pb_text][/et_pb_column][et_pb_column type=”1_3″][et_pb_sidebar admin_label=”Sidebar” orientation=”left” area=”et_pb_widget_area_8″ background_layout=”light” /][/et_pb_column][/et_pb_row][/et_pb_section]