Task: Implement the game of Connect4 in C. Before you start, make sure you understand the game! You can play against a computer or another player at
https://www.cbc.ca/kids/games/all/connect-4
or in plenty of other places on the internet. You can also play against another person with a genuine Connect4™ board!
Academic Honesty: All the code in Connect4 should be written by you. You should not look at a working version of Connect4 in order to write your own code. If you are confused, read the Reasonable / Not Reasonable lists in one of the CS50 assignments.
Getting Started: In the CS50 IDE terminal, execute the following:
wget https://27classrooms.com/wp-content/uploads/2021/10/connect4.zip
And then
unzip connect4.zip
Take a look through the code. The main function has a while loop in it. How would you describe what it does?
Make and run the program as it is – you should see a grid of places for pieces,
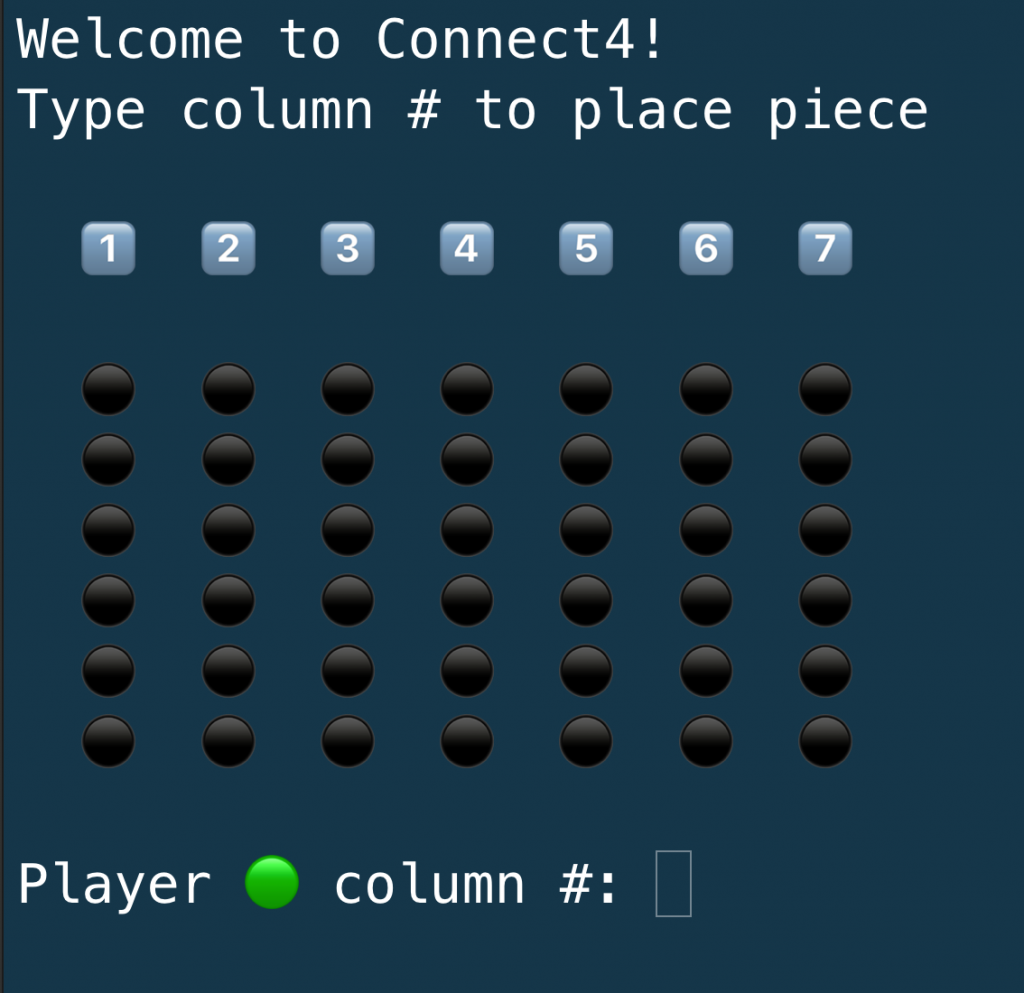
If you try typing a number, nothing happens. But thats because you haven’t written the move function yet! Before you start, make sure you understand the board variable. It is a 2 dimensional array. In move, set board[1][3] to 1.
board[1][3] = 1;
and then make and run connect4 again. What happens? When you can turn any circle of your choice green or purple by writing a line like that, you’re ready to move on.
Move
Before you start, write out the move algorithm in your own words. Make sure you don’t just say “falls to the bottom” but instead discuss changing values in board[][]. Writing the algorithm is part of the assignment, and you’ll be turning this in! Also make a list of cases to test – (i.e. moving in a full column, trying a negative number, etc.)
Then implement move, according to the rules of connect4. In connect4, the player chooses a column, but the board fills from the bottom up. Make sure your code does the right thing (illegal move) when the player tries placing a checker in a space that is already full. When move is complete you should test it! Use the tests you wrote in the previous part. Make sure it works before you move on to the next part.
Win
Once again, write out an algorithm for win in your own words, along with some test cases. (4 in a row up from the bottom, 4 in a row diagonally up, etc). Again, write your algorithm in terms of board[][]. What does 4 in a row look like to a computer?
Then, write the win function in C code. Play a full game against a friend (or enemy).
Tie
It is possible to fill the board but not have a winner. For this one you’ll be adding a NEW function to the program, and also changing the main function to use your new function. Your tie function should return a boolean value, true if the game is over and false if it is not.
Start by writing the algorithm in your own words. It is not enough to say “every space is filled”, because the computer cannot look at all the spaces at once. Then write the tie function and test it. You’ll have to be careful to make sure no one wins! Draw out a full, no-one-winning connect4 board to start. You can actually find pictures of connect4 tie games online if you have trouble.
Video
As practice for the AP Exam task, take a video of you playing your connect4 game. It should be one minute or less in length, and in MP, MOV, AVI or WMV format. Turn it in attached to the Google Classroom assignment, along with your doc showing your written algorithms.
Hard Mode
Finish early? Ready for a more difficult problem? Make a new copy of connect4, named connect4one.c. Make sure you make a new file, because you might not finish this. In that file, write a one player version of connect4, where one player plays against the computer. I recommend you rewrite main so that player1 uses move(int row), and player2 uses a new function, computer_move(void). If the computer moves randomly (but always picks a legal move), ok. But how could the computer be better than that?
At the end, turn in your project with submit50. Turn in the doc with your written algorithms and your video in Google Classroom.
submit50 sobko/checks/connect4